Upload to Firebase Storage
The
Upload
component is a
<input type="file"...
tag with the functionality to upload files to Firebase Storage.
Basic Usage
<script>
import { Upload } from 'sveltekit-fire';
</script>
<Upload
path="path/to/your/folder/file.png"
let:url>
<p>The file has been uploaded!</p>
<a href={url}>Download it here</a>
</Upload>
Preserving file name
If you dont want to overwrite the filename, use the
keepFileName
flag and remove the filename from the
path
<script>
import { Upload } from 'sveltekit-fire';
</script>
<Upload
path="path/to/your/folder"
keepFileName
let:url>
<p>The file has been uploaded!</p>
<a href={url}>Download it here</a>
</Upload>
Error Handling
<script>
import { Upload } from 'sveltekit-fire';
</script>
<Upload
path="path/to/your/folder/file.png"
let:url
let:error>
<p>The file has been uploaded!</p>
<a href={url}>Download it here</a>
<div slot="fallback">
Error uploading file: {error}
</div>
</Upload>
Setting content type
<script>
import { Upload } from 'sveltekit-fire';
</script>
<Upload
path="path/to/your/folder/file.png"
contentType="image/png"
let:url
let:error>
<p>The file has been uploaded!</p>
<a href={url}>Download it here</a>
<div slot="fallback">
Error uploading file: {error}
</div>
</Upload>
Styling the component
All the classes passed to the component are reflected to the
<input type="file"...
tag, so you can pass your own classes or style the button like this if you are using tailwindcss:
<script>
import { Upload } from 'sveltekit-fire';
</script>
<Upload
path="path/to/your/folder/file.png"
contentType="image/png"
let:url
let:error
class="w-full text-sm text-gray-500 file:mr-4 file:py-2 file:px-4 file:rounded-full file:border-0 file:text-sm file:font-semibold file:bg-amber-50 file:text-amber-700 hover:file:bg-amber-100">
<p>The file has been uploaded!</p>
<a href={url}>Download it here</a>
<div slot="fallback">
Error uploading file: {error}
</div>
</Upload>
The above code would produce a button like this:
Custom Upload Buttons
If you don't want to use the browser default input file, you can create your own and pass the
hidden
flag, like this:
<script>
import { Upload } from 'sveltekit-fire';
let inputFile;
</script>
<img on:click={()=>inputFile.click()} src="custom-upload.png" alt="Custom upload" />
<Upload
bind:this={inputFile}
path="path/to/your/folder/file.png"
contentType="image/png"
let:url
let:error
hidden>
<p>The file has been uploaded!</p>
<a href={url}>Download it here</a>
<div slot="fallback">
Error uploading file: {error}
</div>
</Upload>
Showing upload progress
<script>
import { Upload } from 'sveltekit-fire';
</script>
<Upload
path="path/to/your/folder/file.png"
contentType="image/png"
let:url
let:error
let:progress>
<p>The file has been uploaded!</p>
<a href={url}>Download is here</a>
<div slot="fallback">
Error uploading file: {error}
</div>
<div slot="uploading">
{progress}%
</div>
</Upload>
Smooth upload progress with Svelte
<script>
import { Upload } from 'sveltekit-fire';
import { tweened } from 'svelte/motion';
import { cubicOut } from 'svelte/easing';
const animatedProgress = tweened(0, {
duration: 400,
easing: cubicOut
});
animatedProgress.set(0);
</script>
<Upload
path="path/to/your/folder/file.png"
contentType="image/png"
let:url
let:error
let:progress
on:progressChange={(event) => {
animatedProgress.set(event.detail.progress);
}}>
<p>The file has been uploaded!</p>
<a href={url}>Download is here</a>
<div slot="fallback">
Error uploading file: {error}
</div>
<div slot="uploading">
{progress}%
<br />
<div
class="h-6 w-0 rounded z-20 bg-gradient-to-r from-svelte via-amber-600 to-firebase"
style={`width: ${$animatedProgress}%`} />
</div>
</Upload>
The above code will produce a progress indicator like this
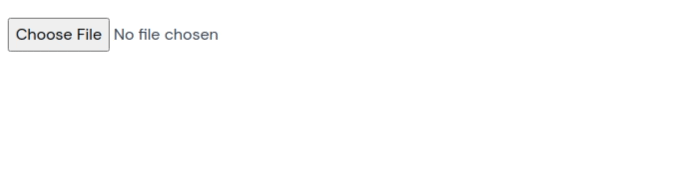
Parameters
Upload props
Props |
Description |
---|---|
path | String | The path to Firebase Storage Folder |
accept | The accepted file types. Default: null |
contentType | The content type metadata to store with the file. Default: do not set any content type |
keepFileName | If true, the file name and extension will be added to the end of path .Default: false |
hidden | If true, the <input type="file"... will have a hidden value. Usefull to create custom upload buttons. Default: false |
log | Display console logs Default: false |
url | Variable where the download url of the file is stored after the upload is complete |
error | Variable where the error message is stored if an error occurs |
progress | Variable where the progress % is stored |
progressMb | Variable where the progress in MB is stored |
Upload slots
Slots |
Description |
---|---|
uploading | Rendered while the file is being uploaded |
fallback | Rendered if an error occurs |
default | Default slot rendered when the upload is complete |
Upload events
Events |
Description |
---|---|
on:progressChange | Dispatched when the progress of the upload changes |
on:uploadComplete | Dispatched when the upload is complete |